- Published on
Crafting Pure Data Collections: Filtering with JavaScript’s .filter( )
Dive into the essentials of JavaScript's .filter() method with this guide. Discover how to refine arrays by filtering out elements based on specific criteria, through practical examples. Ideal for developers aiming to enhance their skills in array manipulation, this post demystifies .filter(), empowering you to write more efficient and readable code.
- Authors
- Name
- pabloLlanes
- @
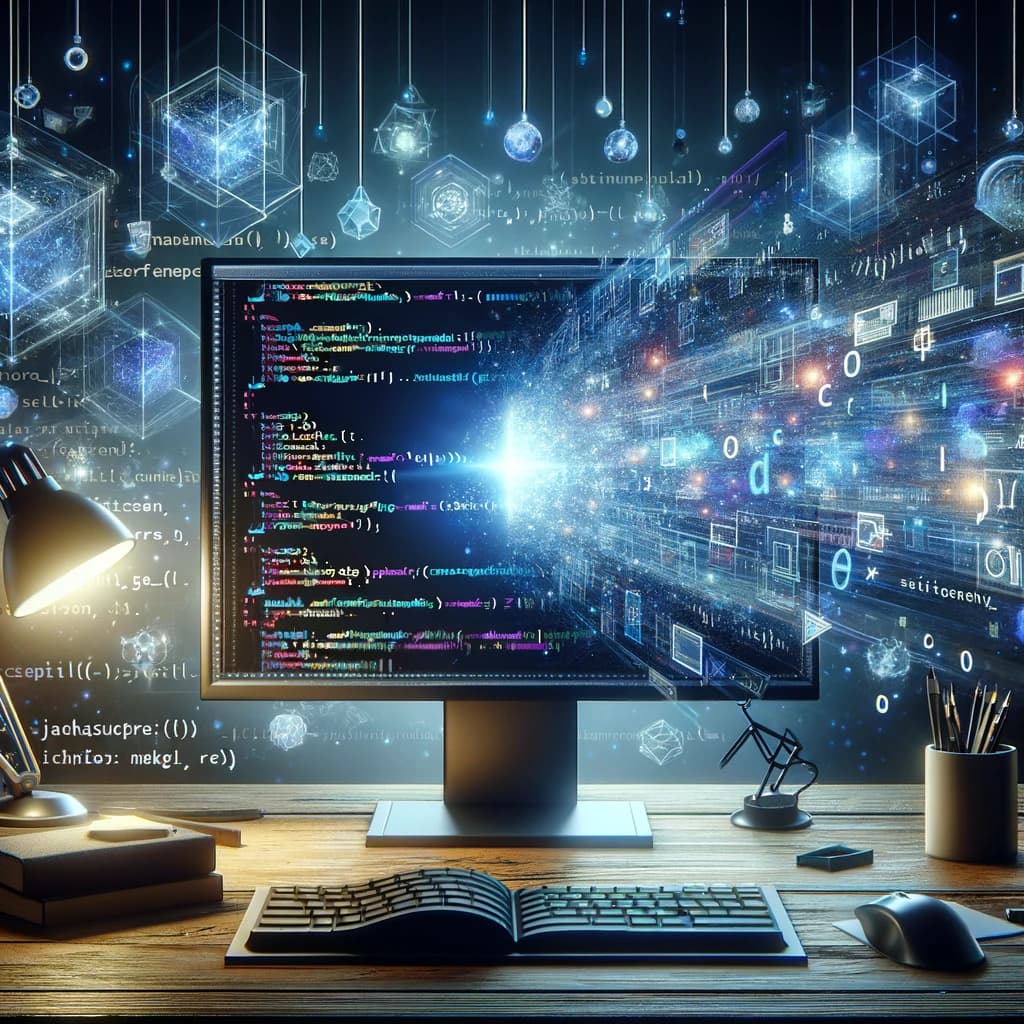
Mastering the JavaScript .filter Method 💧
JavaScript's .filter()
method offers a robust solution for creating a new array filled with elements that pass a test implemented by the provided function. Unlike the .map()
method, which transforms array elements, .filter()
focuses on selecting elements that meet certain conditions, preserving the original array's integrity.
Syntax and Parameters
The .filter()
method syntax is simple and intuitive:
const filteredArray = originalArray.filter(callbackFn, thisArg);
callbackFn
: A function to test each element of the array. It returnstrue
to keep the element,false
otherwise. It accepts three arguments:element
: The current element being processed.index
: The index of the current element.array
: The array.filter()
was called upon.
thisArg
(Optional): An object to use asthis
when executingcallbackFn
.
How It Works
const filteredArray = originalArray.filter((element, index, array) => {
// Condition for element inclusion
return condition;
});
Key Points
- Selection Based on Condition:
.filter()
selects elements that satisfy the condition specified incallbackFn
. - Non-mutating Method: It creates a new array and does not modify the original array.
- Use Case: Ideal for removing unwanted elements from an array based on a condition.
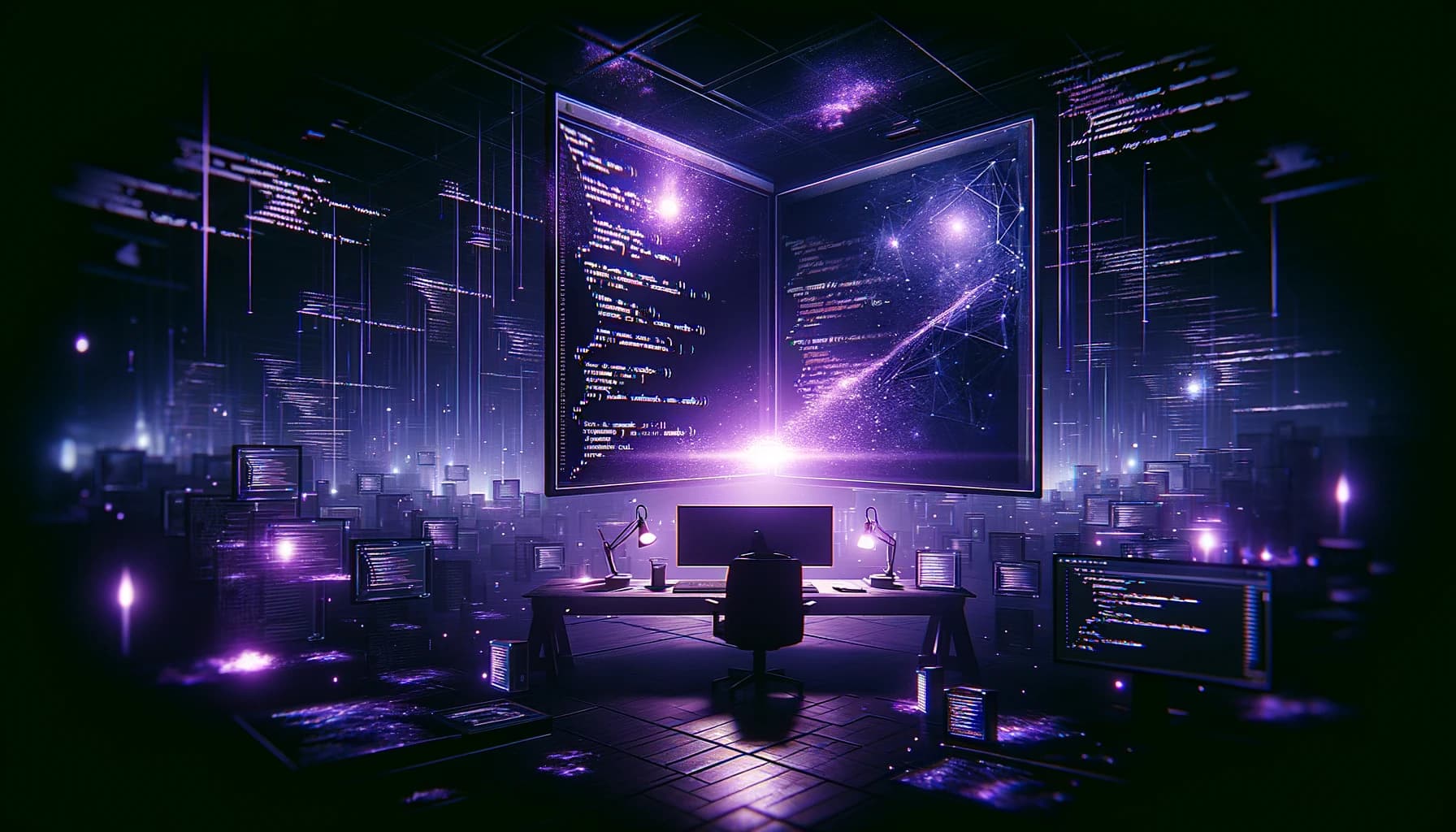
Practical Examples 🛠️
1- Filtering Even Numbers
// Array of numbers
const numbers = [1, 2, 3, 4, 5, 6]
// Filter even numbers
const evenNumbers = numbers.filter(number => number % 2 === 0);
console.log(evenNumbers);
// Output: [2, 4, 6]
2- Filtering Specific Characters
// Array of characters from a movie
const characters = [
{ name: 'Aldo Raine', faction: 'allies' },
{ name: 'Hans Landa', faction: 'enemys' },
{ name: 'Hugo Stiglitz', faction: 'allies' },
{ name: 'Donny Donowitz', faction: 'allies' },
{ name: 'Fredrick Zoller', faction: 'enemys' }
];
// Filter characters by faction using arrow function
const alliesWithArrow = characters.filter(character => character.faction === 'allies');
console.log(alliesWithArrow);
// Output: [
// { name: 'Aldo Raine', faction: 'allies' },
// { name: 'Hugo Stiglitz', faction: 'allies' }
// { name: 'Donny Donowitz', faction: 'allies' }
// ]
// Filter characters by faction using traditional function
const enemysWithoutArrow = characters.filter(function(character) {
return character.faction === 'enemys';
});
console.log(enemysWithoutArrow);
// Output: [
// { name: 'Hans Landa', faction: 'enemys' },
// { name: 'Fredrick Zoller', faction: 'enemys' }
// ]
3- Removing Falsy Values
// Array with mixed falsy and truthy values
const mixedValues = [0, "Hello", false, 19, '', 'JavaScript', null]
// Filter out falsy values
const truthyValues = mixedValues.filter(value => value);
console.log(truthyValues);
// Output: ["Hello", 19, 'JavaScript']
4- Filtering Senior Players from 'Paris Saint-Germain'
const footballPlayers = [
{ name: 'Lionel Messi', age: 34, team: 'Paris Saint-Germain' },
{ name: 'Cristiano Ronaldo', age: 37, team: 'Manchester United' },
{ name: 'Kylian Mbappé', age: 23, team: 'Paris Saint-Germain' },
{ name: 'Erling Haaland', age: 21, team: 'Borussia Dortmund' },
{ name: 'Neymar Jr', age: 29, team: 'Paris Saint-Germain' },
{ name: 'Jadon Sancho', age: 21, team: 'Manchester United' }
];
// Filter players over 23 years old who play for 'Paris Saint-Germain'
const psgSeniorPlayers = footballPlayers.filter(player => player.age > 23 && player.team === 'Paris Saint-Germain');
console.log(psgSeniorPlayers);
// Output:
// [
// { name: 'Lionel Messi', age: 34, team: 'Paris Saint-Germain' },
// { name: 'Neymar Jr', age: 29, team: 'Paris Saint-Germain' }
// ]
// First, filter players over 23 years old who play for 'Paris Saint-Germain'
// Then, map the resulting array to extract and transform the data
const psgSeniorPlayerNames = footballPlayers
.filter(player => player.age > 23 && player.team === 'Paris Saint-Germain')
.map(player => `${player.name} is ${player.age} years old`);
console.log(psgSeniorPlayerNames);
// Output:
// ["Lionel Messi is 34 years old", "Neymar Jr is 29 years old"]
// or alternatve using reusable filtering function
function isSeniorPSGPlayer(player) {
return player.age > 23 && player.team === 'Paris Saint-Germain';
}
// Using the filtering function with .filter() and then transforming with .map()
const psgSeniorPlayerNames = footballPlayers
.filter(isSeniorPSGPlayer)
.map(player => `${player.name} is ${player.age} years old`);
console.log(psgSeniorPlayerNames);
// Output:
// ["Lionel Messi is 34 years old", "Neymar Jr is 29 years old"]
Conclusion
The .filter()
method is indispensable for developers looking to perform complex data manipulation tasks with ease. By mastering .filter()
, you unlock the potential to write cleaner, more efficient JavaScript code. Experiment with .filter()
in your projects, and see firsthand how it can streamline your array processing workflows.
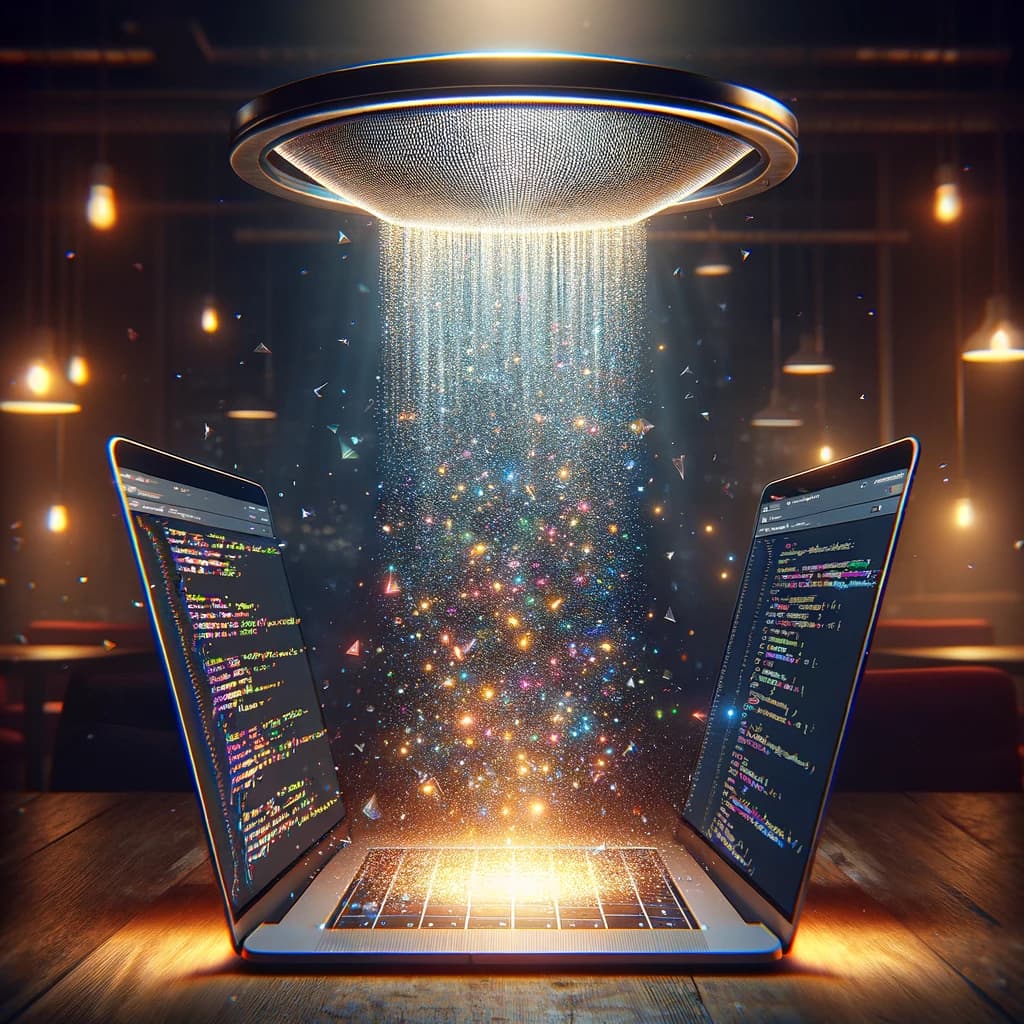
Embrace the power and versatility of .filter()
in your JavaScript endeavors, refining arrays with elegance and precision.