- Published on
JavaScript’s .forEach(): Embracing Array Iteration with Purpose
Dive into the essentials of JavaScript's .forEach() method with this detailed guide. Discover how to effectively iterate over arrays for side effects, from logging elements to applying functions directly. Ideal for developers aiming to enhance their understanding of array iteration, this article demystifies .forEach(), making iteration over arrays more straightforward and efficient.
- Authors
- Name
- pabloLlanes
- @
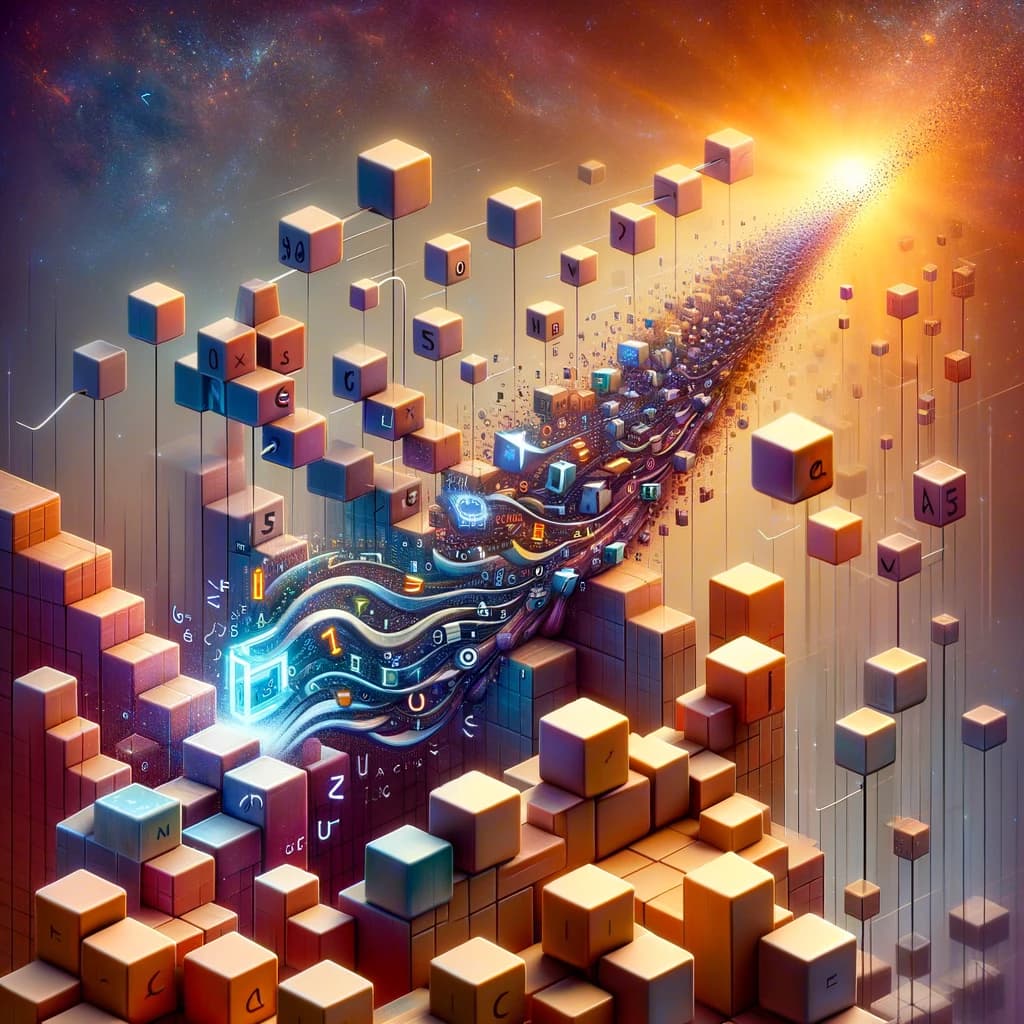
Mastering the JavaScript .forEach Method💡
The JavaScript forEach()
method is an essential tool for iterating over arrays to apply a function on each element, allowing side effects. Unlike the map()
method, forEach()
does not return a new array but instead is used for operations that result in a side effect. Here's an overview of its syntax, parameters, and practical usage.
Syntax and Parameters
The forEach()
method is utilized as follows:
array.forEach(callbackFn, thisArg);
callbackFn
: A function to execute on each element in the array. It accepts up to three arguments:element
: The current element being processed.index
: The index of the current element.array
: The arrayforEach()
was called upon.
thisArg
(Optional): An object to use asthis
when executingcallbackFn
.
How It Works
array.forEach((element, index, array) => {
// Operation to perform on each element
});
Key Points
- Side Effects: Ideal for operations that need to perform a function on each element of an array without needing a returned array.
- No Return Value:
forEach()
returnsundefined
and is used for its side effects. - Direct Manipulation: It can be used to directly manipulate or modify an array.
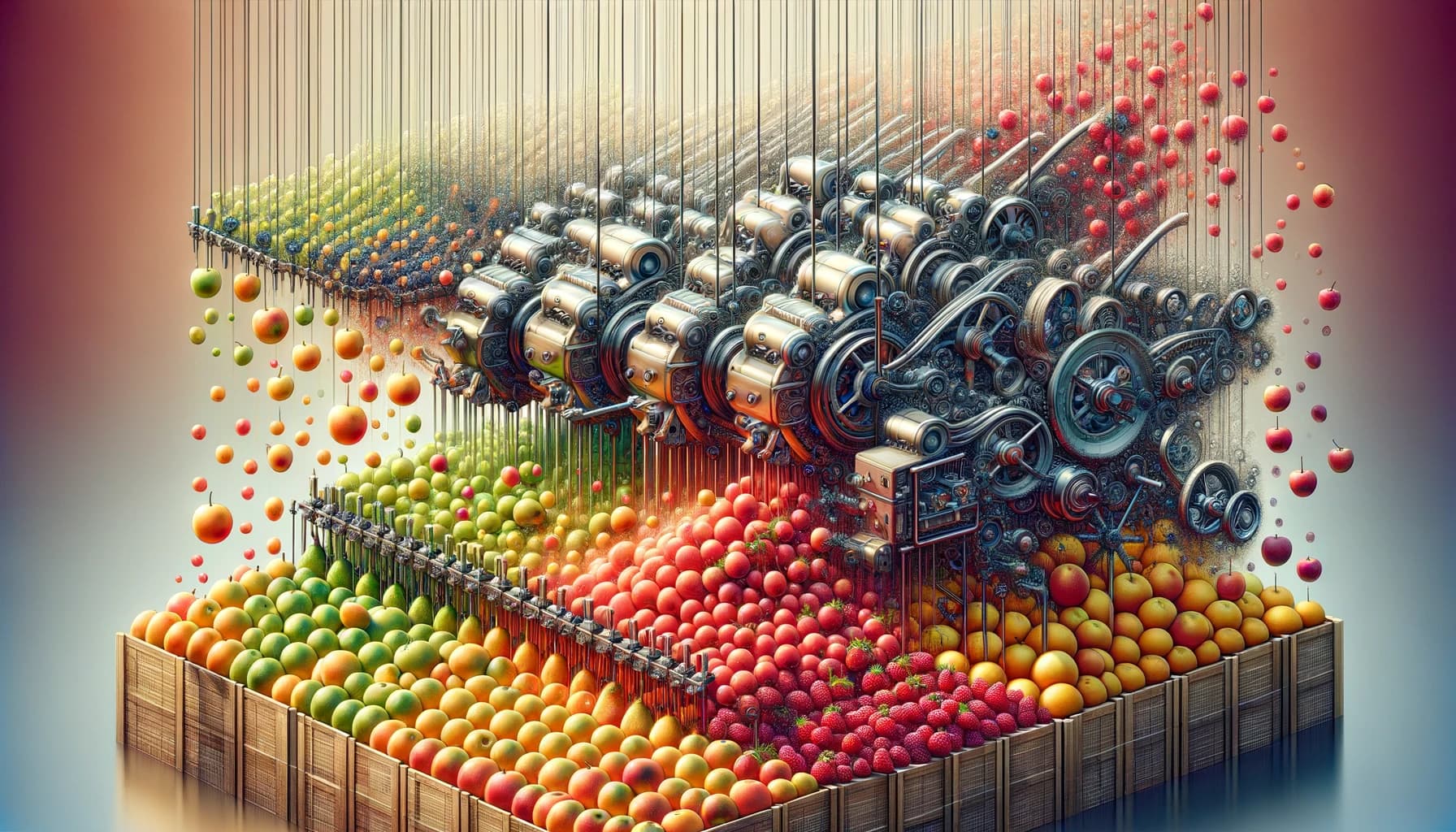
Examples 🖥️
1- Logging Array Elements
const fruits = ['Apple', 'Banana', 'Cherry'];
fruits.forEach((fruit, index) => {
console.log(index, fruit);
});
// Output:
// 0 'Apple'
// 1 'Banana'
// 2 'Cherry'
2- Applying Functions to Array Elements
const numbers = [1, 2, 3, 4, 5];
const double = (number) => console.log(number * 2);
numbers.forEach(double);
// Output:
// 2
// 4
// 6
// 8
// 10
3- Updating Array Elements
let amounts = [100, 200, 300, 400, 500];
amounts.forEach((amount, index, arr) => {
arr[index] = amount + 100;
});
console.log(amounts);
// Output:
// [200, 300, 400, 500, 600]
Updating Objects within an Array
Imagine managing a list of tasks where each task is an object containing a description
and a completed
status.
const tasks = [
{ description: 'Finish writing article', completed: false },
{ description: 'Review pull request', completed: false },
{ description: 'Update documentation', completed: false },
];
tasks.forEach(task => {
task.completed = true; // Mark each task as completed
});
console.log(tasks);
Aggregating Data from an Array of Objects
Suppose you have an array of products, each with a name
and price
, and you want to calculate the total price.
const products = [
{ name: 'Keyboard', price: 30 },
{ name: 'Mouse', price: 10 },
{ name: 'Monitor', price: 150 },
];
let totalPrice = 0;
products.forEach(product => {
totalPrice += product.price;
});
console.log(`Total Price: $${totalPrice}`);
Filtering and Applying Operations
Consider a scenario where you need to filter an array based on certain criteria and then perform operations on the filtered elements.
const numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
const evenNumbers = [];
numbers.forEach(number => {
if (number % 2 === 0) {
evenNumbers.push(number * 2); // Double each even number
}
});
console.log(evenNumbers);
Handling Nested Arrays
Working with nested arrays can be complex. Here's how you can use forEach
to simplify the process.
const matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9],
];
matrix.forEach(row => {
row.forEach(element => {
console.log(element); // Log each element of the matrix
});
});
These examples illustrate the flexibility and power of the .forEach()
method in handling arrays and object collections. By integrating forEach
into your JavaScript projects, you can perform array manipulations more effectively and write cleaner, more readable code.
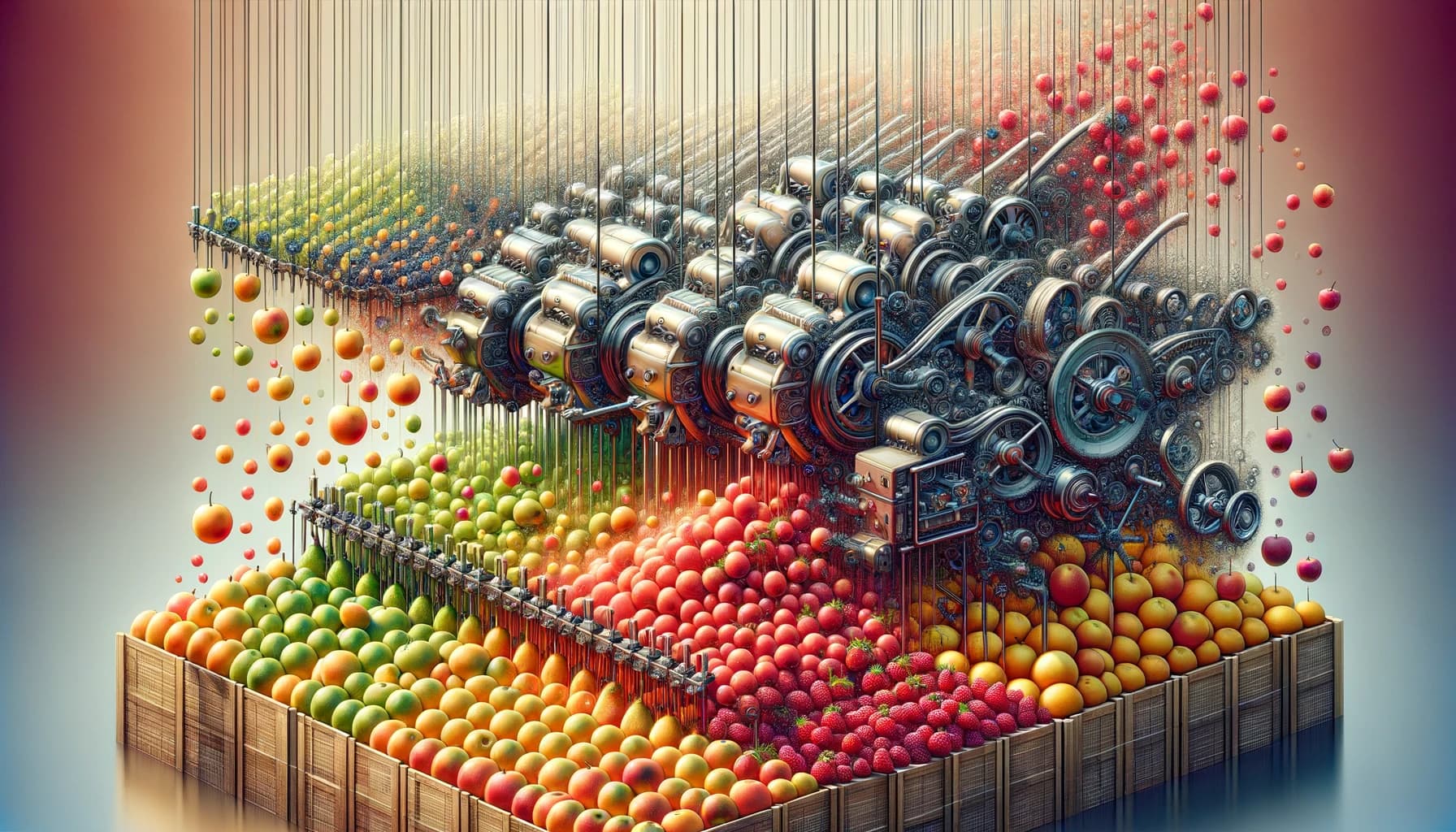
Leverage the .forEach() method in your JavaScript endeavors for efficient array iteration and manipulation, enhancing the overall functionality of your code without the necessity of a return value.