- Published on
Transforming Data with Elegance: The JavaScript .map( ) Mastery
Embark on a transformative journey with JavaScript's .map() method, where data manipulation becomes not just a task, but an art. This guide unveils the secret to elegantly reshaping arrays, from amplifying numbers to weaving rich narratives around simple data structures. Discover through vivid examples how .map() elevates coding from mere functionality to creative expression. Perfect for both budding and veteran developers, this piece not only clarifies the .map() method but inspires a refined approach to programming. Dive in, and let each line of code you write thereafter be a stroke of genius.
- Authors
- Name
- pabloLlanes
- @
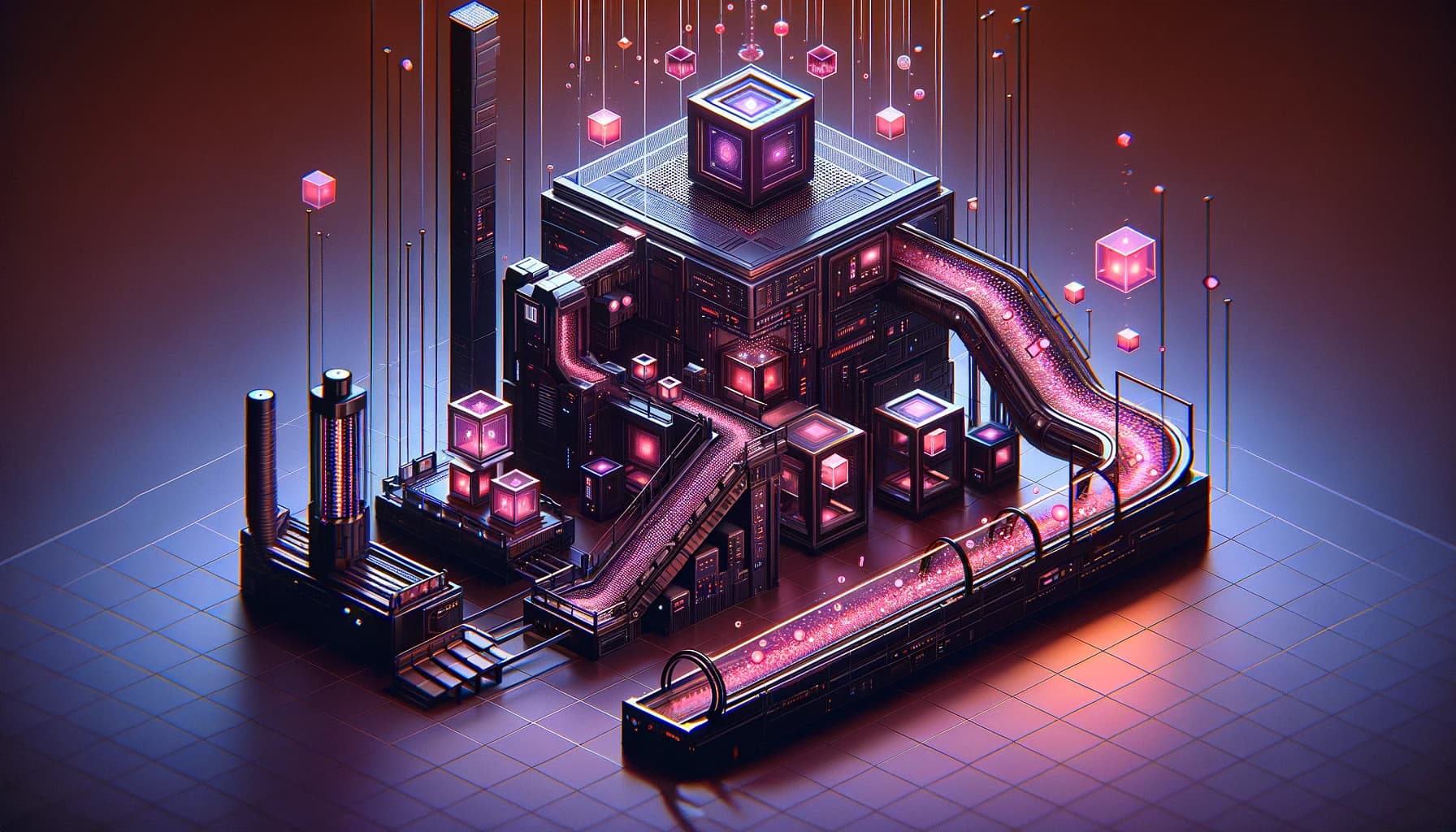
Understanding the JavaScript .map Method💡
JavaScript's map()
method is a powerful tool for transforming arrays without altering the original array. This method creates a new array by applying a function to each element of the original array. Here's a simplified breakdown of its syntax, parameters, and benefits.
Syntax and Parameters
The map()
method is used as follows:
const newArray = originalArray.map(callbackFn, thisArg);
callbackFn
: A function executed for each element in the array, transforming each element into a new value. It can take three arguments:element
: The current element being processed in the array.index
: The index of the current element.array
: The arraymap()
was called upon.
thisArg
(Optional): An object to use asthis
when executingcallbackFn
.
How It Works
const newArray = originalArray.map((element, index, array) => {
// Transformation logic here
return transformedValue;
});
Key Points
- Transformation: The
callbackFn
is your transformation logic applied to each element. - Return Value: A new array, with each element being the result of the
callbackFn
. - Usage Note: Since
map()
constructs a new array, it should be used when the transformed array is needed. If no new array is needed,forEach
or afor...of
loop might be more appropriate.
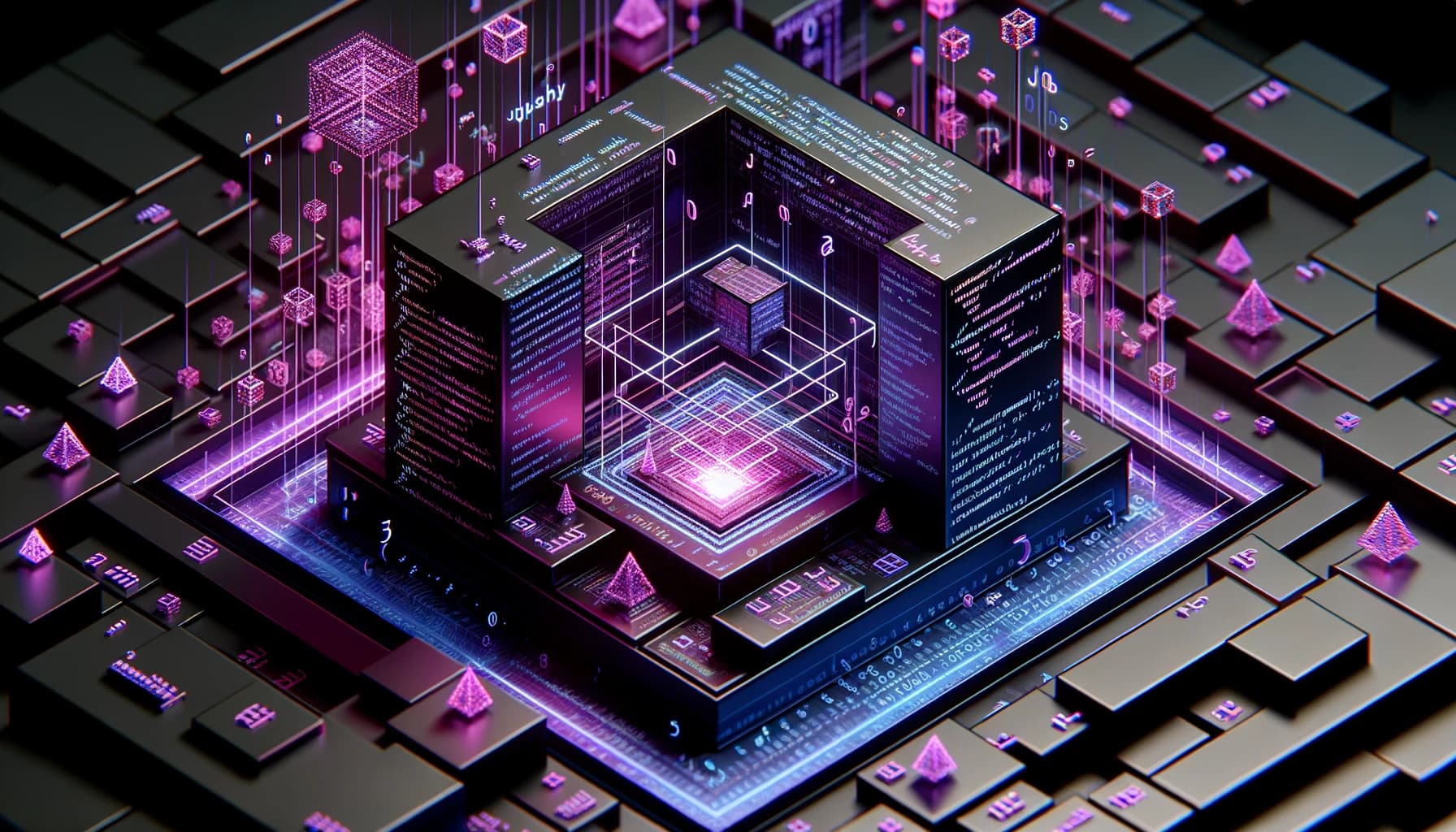
Examples 🖥️
1- Doubling Numbers
// Our list of numbers
const numbers = [1, 2, 3, 4, 5]
// Doubling each number using .map() with a standard function
const doubledNumbers = numbers.map(function (number) {
return number * 2
})
console.log(doubledNumbers)
// Output:
// [2, 4, 6, 8, 10]
2- Prefixing Rock Bands with "The"
// Our rock band list
const rockBands = ['Who', 'Cure', 'Rolling Stones', 'Beatles', 'Clash']
// Adding "The" using .map() with a arrow function
const bandsWithThe = rockBands.map((band) => 'The ' + band)
console.log(bandsWithThe)
// Output:
// ['The Who', 'The Cure', 'The Rolling Stones', 'The Offpring', 'The Clash']
3- Describing characters with Hobbies
// Array of objects representing characters from the Simpsons
const theSimpsonsArray = [
{ name: 'Homer', surname: 'Simpson', hobby: 'Bowling' },
{ name: 'Marge', surname: 'Simpson', hobby: 'Painting' },
{ name: 'Bart', surname: 'Simpson', hobby: 'Skateboarding' },
{ name: 'Lisa', surname: 'Simpson', hobby: 'Playing Saxophone' },
{ name: 'Maggie', surname: 'Simpson', hobby: 'Pacifier' },
]
// Use map to create a new array with descriptions of each character's hobby
const characterDescriptions = theSimpsonsArray.map(function (character) {
// Return a string for each character combining their name and hobby
return character.name + ' loves ' + character.hobby + '.'
})
console.log(characterDescriptions)
// Output:
// [
// 'Homer loves Bowling.',
// 'Marge loves Painting.',
// 'Bart loves Skateboarding.',
// 'Lisa loves Playing Saxophone.',
// 'Maggie loves Pacifier.'
// ]
// Use map with an arrow function to create a new array of just the character names
const characterNames = theSimpsonsArray.map((character) => character.name)
console.log(characterNames)
// Output:
// ['Homer', 'Marge', 'Bart', 'Lisa', 'Maggie']
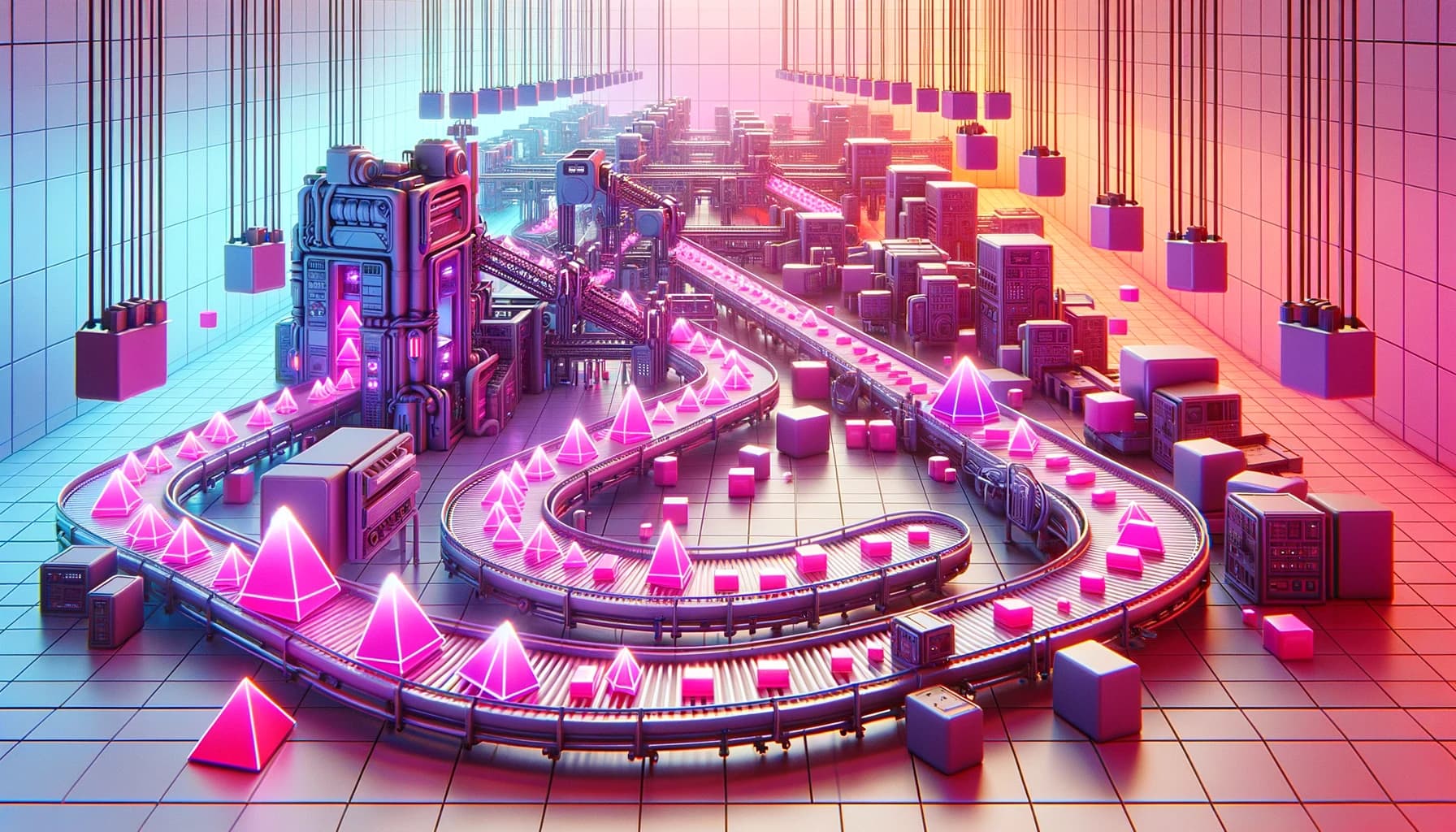
Embrace the power of .map() in your projects, and watch how it transforms not just your arrays, but also the way you think about and solve programming challenges.